Almost in every application that we develop, we need to send some kind of notification. Laravel comes with a very nice responsive template for emails that we use a lot of times without changing much of it.
Basics
Creating a Notification is as easy as executing the following command
php artisan make:notification InvoicePaid
This will create a file in the `app/notifications` folder.
To send a notification we use this code
Notification::send($users, new InvoicePaid($invoice));
// or
$user->notify(new InvoicdPaid($invoice);
MailMessage
The basic structure and functionality that we use most of the time are as follows.
/**
* Get the mail representation of the notification.
*
* @param mixed $notifiable
* @return \Illuminate\Notifications\Messages\MailMessage
*/
public function toMail($notifiable)
{
$url = url('/invoice/'.$this->invoice->id);
return (new MailMessage)
->greeting('Hello!')
->line('One of your invoices has been paid!')
->action('View Invoice', $url)
->line('Thank you for using our application!');
}
Probably you already know but we can publish the HTML and Text resources.
php artisan vendor:publish --tag=laravel-notifications
// and
php artisan vendor:publish --tag=laravel-mail
Tips and Tricks
In the past section we took a look at what is the basic an normal situation creating an Email Notification.
Let's see all the options that MailMessage provides and how to use them.
- greeting(string $text)
- salutation(string $text)
- line(\Illuminate\Contracts\Support\Htmlable|string|array $text)
- success()
- error()
- level('info') //'info','success','error'
- subject(string $text)
- action(string $text, string $url)
Example
/**
* Get the mail representation of the notification.
*
* @param mixed $notifiable
* @return \Illuminate\Notifications\Messages\MailMessage
*/
public function toMail($notifiable)
{
return (new MailMessage)
->subject('Lorem ipsum')
->greeting('Greeting Victor Yoalli!')
->salutation(new HtmlString('<strong>Salutation</strong> Victor Yoalli!'))
->line('Line of Plain Text')
->with(new HtmlString('<b>new HtmlString</b>("using with.")'))
->action('Notification Action', url('/'))
->line(new HtmlString(‘<b>new HtmlString</b>("using <i>line</i>.")'))
->line(['array of elements:[','uno','dos','tres',']using line'])
->level('info')
->error()
->success()
->line('Thank you for using our application!');
}
As you you can see in the result the:
- subject() function is not show because is the email subject.
- greeting() will always be shown at the beginning no matter where you call this function.
- salutation() is similar to greeting but it will be shown at the end.
- line() accepts 3 type of data: string, HtmlString and array(), I use the three cases in this example.
- with() is similar to line()
- action() prints a call to action button and it can only be one. If you try to add more than one, the last one will be displayed.
- error() will change the color red, success() to green of the action() button, the default is blue which is the same as if you use level(‘info’).
- level() have the same effect, except that you need to specify 'info','error' or 'success'.
Result
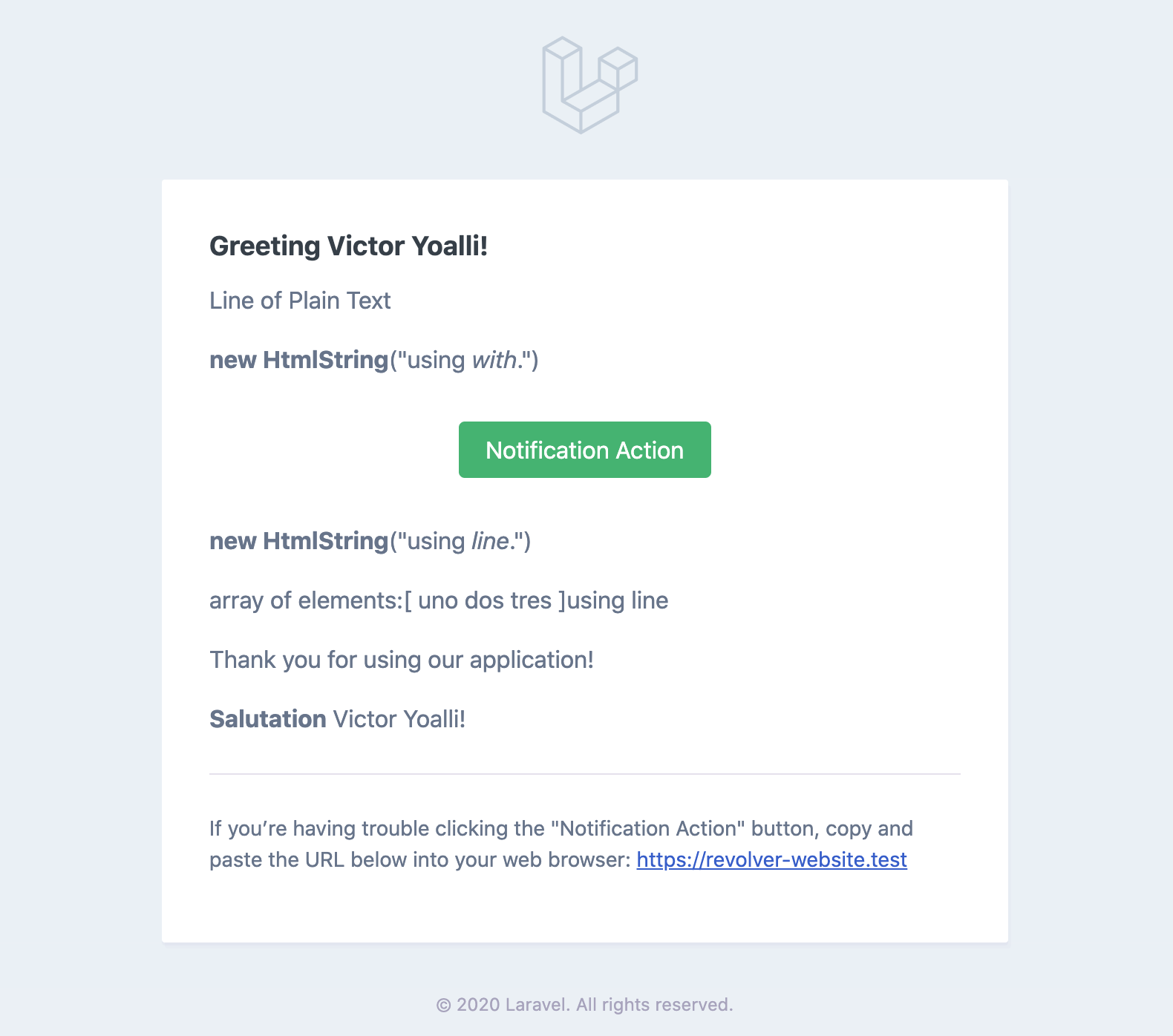
Laravel Email Notification Example
I hope it helps. :)